Imagine a number, any number. Now, before that number slips from your mind, let’s store it for the future. When you conceive of a number, you’re essentially holding onto its value mentally. However, to recall it later – particularly if your memory resembles mine – you jot it down on a piece of paper. And for matters of great significance, you’d secure it in a safe location. In the realm of computer science, this secure location is termed a ‘variable.’ They earn this moniker because, well, they’re ‘capable of being altered or modified.’ You have the freedom to assign a name to a variable, choosing nearly any label you desire, and you possess the authority to alter the value at will.
For instance, I had the number 5 in mind. Therefore, at the Python command prompt, I will establish a variable bearing the witty title ‘number,’ and proceed to input my value:
>>> number = 5
Let’s temporarily set aside that number for now. We’ll revisit it shortly. If you’re new here, you might find it beneficial to begin from the start by checking out our introduction to the Python programming language.
Python Variable Assignment with Historical Figures
In the preceding example, Attlee, Truman, and Stalin are individually contemplating numbers. We can liken their names to variables and the numbers they have in mind to values, as follows:
>>> Attlee = 7
>>> Truman = 101
>>> Stalin = “a number”
This is referred to as an assignment statement. We employ the equal sign (=) to assign a value to a variable. It’s akin to stating, “Attlee equals 7”. If I wish to ascertain the number Attlee has in mind, I can easily input his name at the command prompt:
>>> Attlee
7
Now it’s your chance. Give it a try with the remaining heads of state.
>>> Truman
101
>>> Stalin
‘a number’
Stalin is being a bit playful here. ‘a number’ is actually a string, not a numerical value, and we haven’t discussed strings yet. But you can modify the value of a variable by assigning it a new value. Let’s go ahead and change Stalin’s value to a floating-point number. How about using the value of pi?
>>> Stalin = 3.14
>>> Stalin
3.14
I have quite a few values occupying my thoughts, making it challenging to retain anything beyond 3.14. Fortunately, there’s a straightforward solution: we can effortlessly assign the value of pi to a variable by making use of Python’s math module.
>>> import math
>>> math.pi
3.141592653589793
>>> Stalin = math.pi
>>> Stalin
3.141592653589793
As simple as can be.
Understanding Variable Naming Rules and Conventions
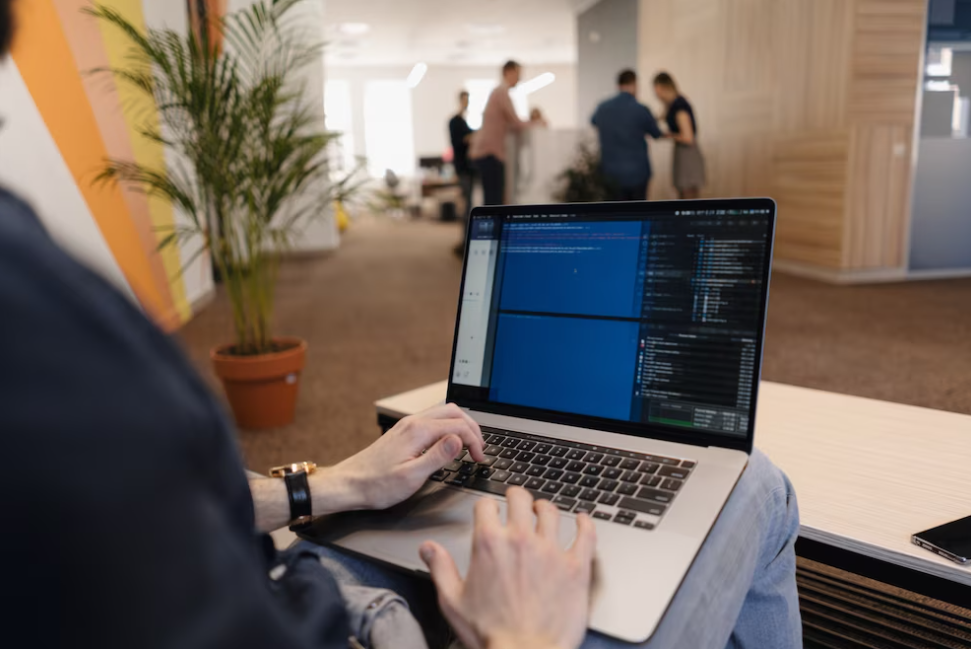
You might already be acquainted with algebraic equations, like the quadratic example:
ax² + bx + c = 0
In mathematics, variables typically take the form of single letters like x, y, and z, or they may even utilize Greek symbols such as π or θ. Mathematicians frequently employ variables when they are uncertain about a specific value but are actively striving to determine it. However, the approach is somewhat distinct in Python. In Python, you are obligated to provide a value to a variable before you can make use of it, whether that value is zero or an empty placeholder. For instance, if I were to mention the variable “Guido” before assigning it a value:
>>> Guido
The following error message will be generated:
Traceback (most recent call last):
File “<stdin>”, line 1, in <module>
NameError: name ‘Guido’ is not defined</module></stdin>
Variable assignment operates from left to right.
>>>> Guido = 0
That is permissible as it stands.
>>> Guido = “”
And furthermore:
>>>> Guido = False
However, attempting the following will result in a troublesome error.
>>> 0 = Guido
>>> ‘’ = Guido
>>> False = Guido
The initial two attempts resulted in an error message stating:
File “<stdin>”, line 1
SyntaxError: can’t assign to literal</stdin>
However, the final attempt led to an error message stating:
File “<stdin>”, line 1
SyntaxError: assignment to keyword</stdin>
This occurs because “False” is a reserved word in Python. It falls under the category of Boolean values in computer science, and you cannot employ it as a variable name. Python includes a module named “keyword,” which contains a function called “kwlist.” When you import the “keyword” module and invoke “kwlist,” it will furnish you with a list containing Python’s reserved keywords. Give it a try:
>>>> import keyword
>>> keyword.kwlist
These are all the terms you should avoid using as variable names. However, that’s perfectly fine because when it comes to choosing variable names, you have a wide range of possibilities. Well, almost. There are specific rules you must adhere to and some recommended conventions you should consider.
The Guidelines
Variable names must commence with either a letter or an underscore, like:
_underscore
underscore_
The remaining part of your variable name can encompass letters, numbers, and underscores.
password1
n00b
underscores
It’s important to note that variable names are case-sensitive, which means that `casesensitive`, `CASESENSITIVE`, and `Case_Sensitive` represent distinct variables.
The Guidelines for Readability
Clarity and readability are of utmost importance. Which of the following options do you find the easiest to read? I’m hoping you’ll choose the first example.
python_puppet
pythonpuppet
pythonPuppet
Descriptive Names for Clarity
Meaningful and descriptive names are highly beneficial. If you were developing a program to tally all the bad puns within this book, which variable name do you believe is superior?
totalbadpuns
super_bad
Avoiding Confusing Characters
It’s wise to refrain from using lowercase ‘l,’ uppercase ‘O,’ and uppercase ‘I.’ Why? Because ‘l’ and ‘I’ closely resemble the numeral 1, while ‘O’ bears a striking resemblance to 0.
Conclusion
Mastering Python variable assignment is essential for effective programming. This article has explored the fundamentals of Python variable management, from assigning values to variables to understanding the rules and conventions for naming them. By adhering to best practices, such as choosing descriptive names and avoiding confusing characters, you can greatly enhance your code’s readability and maintainability. Python’s flexibility in naming variables allows for creative and meaningful labeling, empowering developers to express their intentions clearly. So, whether you’re a beginner or an experienced coder, following these guidelines will help you write cleaner and more efficient Python code.