Open your mental pantry, and inside, you’ll discover an array of food storage options. There are cardboard boxes, Ziplocs, Tupperware containers, plastic bags securely fastened with twist ties, and, at the heart of our discussion, storage jars. Now, imagine computer memory as a pantry filled with these storage jars. When working with Python, you essentially place values into these jars and affix a label, or variable, to each one, enabling you to locate your stored values later on.
Consider the storage jar as a designated memory space where I intend to store my beloved peanut butter and jelly. For instance, let’s say I have a value representing peanut butter, denoted as 5. We can employ the id() function to reveal the precise memory address where this value resides.
>>> id(5)
10188960
Imagine that lengthy number as a storage jar, a container where we can store something valuable.
Now, I have the ability to attach a label to this jar:
>>> peanut_butter = 5
>>> peanut_butter
5
To determine the whereabouts of the value linked to ‘peanut_butter,’ I can utilize the ‘id()’ function by passing my variable as an argument.
>>> id(peanut_butter)
10188960
Additionally, it’s possible to affix multiple labels to the same jar:
>>> crunchy = 5
>>> crunchy
5
And verify its memory location:
>>> id(crunchy)
10188960
We have the capability to assign variables to other variables. So, let’s begin by modifying the value of ‘crunchy’ first:
>>> crunchy = 7
>>> peanut_butter = crunchy
>>> peanut_butter
7
>>> crunchy
7
Observe the outcome when we revert ‘crunchy’ back to the value of 5.
>>> crunchy = 5
>>> crunchy
5
>>> peanut_butter
7
Our ‘peanut_butter’ variable remained unaffected. This is because when we assigned it to ‘crunchy’ in this manner:
>>> peanut_butter = crunchy
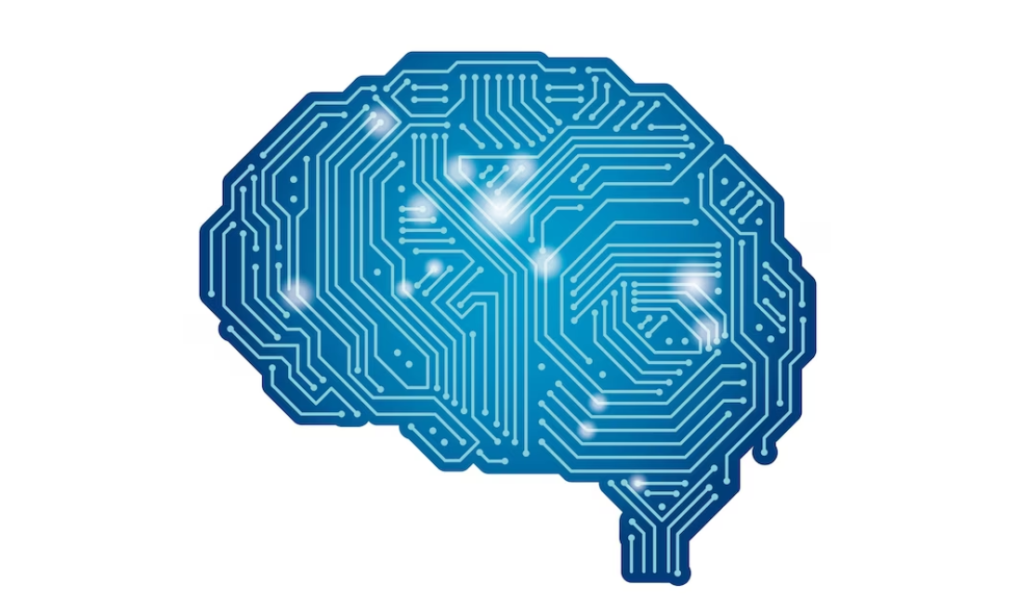
We assigned it the value associated with ‘crunchy,’ which happened to be 7. Now, the question arises: Where on the shelf can we find our storage jar containing the value 7?
>>> id(peanut_butter)
10189024
Imagine I have a storage jar containing five scoops of peanut butter:
>>> pb = 5
Typically, jelly is stored in a smaller container. Let’s assume I have three scoops of it:
>>> j = 3
What is the total count of peanut butter and jelly scoops in my possession?
>>> pb + j
8
Now, let’s assign that statement to a variable.
>>> pbj = pb + j
>>> pbj
8
All these delectable food analogies are quite appetizing. How about we satisfy our hunger by crafting a delicious sandwich? To prepare it, we’ll employ two scoops of peanut butter and one scoop of jelly. To achieve this, we’ll need to update our variables using the subtraction operator, as demonstrated below:
>>> pb = pb – 2
>>> pb
3
>>> j = j – 1
>>> j
2
So, what’s the remaining quantity of peanut butter and jelly at our disposal?
>>> pbj = pb + j
>>> pbj
5
Conclusion
In summary, understanding Python’s variable assignment and memory handling is akin to organizing a pantry. Just as you label jars to find ingredients easily, Python associates variables with values, enabling efficient data manipulation. The `id()` function acts as a memory address, akin to identifying a jar. You can assign multiple variables to the same value, highlighting Python’s memory optimization. Importantly, modifying one variable doesn’t affect others, ensuring data integrity. With this grasp of Python’s inner workings, you can write more efficient and robust code, enhancing your programming skills.