In the realm of programming, Python has consistently proven itself as a versatile and dynamic language. One of its powerful features is the ability to manipulate data structures with ease. Lists, in particular, are a fundamental data structure that Python offers, allowing programmers to store and manage collections of items. But did you know that Python lists can be multiplied? That’s right! In this article, we’ll delve into the world of Python list multiplication, exploring its mechanics, use cases, and benefits.
Understanding Python List Multiplication
Python list multiplication might sound like a complex concept, but in reality, it’s quite intuitive. At its core, list multiplication involves repeating the elements of a list a certain number of times to create a new list. This operation is achieved using the * operator.
Here’s a simple example to illustrate the concept:
original_list = [1, 2, 3] multiplied_list = original_list * 3 print(multiplied_list) |
In this example, the original list [1, 2, 3] is multiplied by 3, resulting in the multiplied_list containing [1, 2, 3, 1, 2, 3, 1, 2, 3]. Python intelligently duplicates the elements in the list the specified number of times.
Python List Multiplication in Action
List multiplication can be incredibly useful in various programming scenarios. Here are some common use cases where list multiplication shines:
Initializing Lists
List multiplication can be employed to create lists with repeated initial values. For instance, if you want to create a list of zeros of a certain length, you can achieve this easily:
zero_list = [0] * 5 # Creates [0, 0, 0, 0, 0] |
Generating Patterns
You can use list multiplication to generate patterns or sequences of values. Consider creating a list of consecutive odd numbers:
odd_numbers = [1, 3, 5] * 4 # Generates [1, 3, 5, 1, 3, 5, 1, 3, 5, 1, 3, 5] |
String Manipulation
Python lists aren’t limited to containing only numbers. They can also hold strings, making list multiplication useful for string manipulation:
repeated_string = [“Hello”] * 3 # Produces [‘Hello’, ‘Hello’, ‘Hello’] |
Nested Lists
List multiplication also works with nested lists, which are lists containing other lists. This can be particularly helpful in creating 2D grids or matrices:
grid = [[0] * 3 for _ in range(4)] # Generates a 4×3 grid of zeros |
Benefits of Using List Multiplication
Python list multiplication offers several advantages that can simplify your code and enhance your programming experience:
- Conciseness: List multiplication allows you to create repeated patterns or sequences with just a single line of code. This conciseness can make your code more readable and maintainable.
- Efficiency: When compared to traditional loop-based methods, list multiplication can be more efficient for creating lists with repeated values. Python’s underlying implementation optimizes this process.
- Ease of Use: List multiplication is straightforward and easy to understand, especially for those familiar with basic arithmetic operations.
Best Practices and Considerations
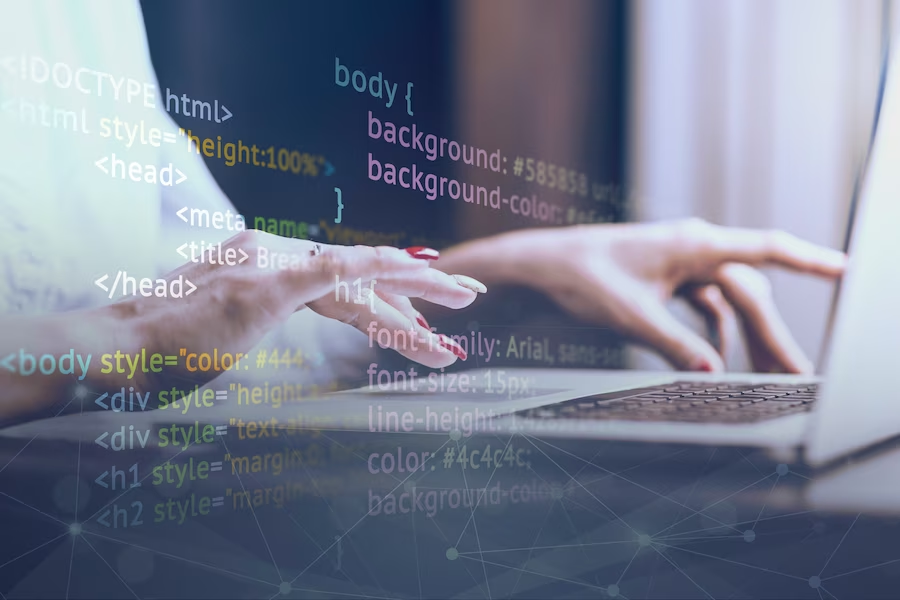
List multiplication is a powerful technique in programming that allows you to create repeated copies of elements within a list. However, like any tool, there are important best practices and considerations to keep in mind in order to use it effectively and avoid potential pitfalls.
Immutable Elements
When utilizing list multiplication, it’s crucial to understand how it interacts with mutable objects, such as lists. List multiplication doesn’t create new instances of the elements within the list; rather, it repeats references to the same elements. This can lead to unexpected side effects if you’re not careful. Modifying an element in one part of the list can impact the entire list due to the shared references. To avoid unintended behavior, it’s important to consider the immutability of the elements you’re working with.
Use Cases
List multiplication is most beneficial when you need to create a list containing repeated copies of the same element. For instance, if you want to initialize a list with a certain number of default values, list multiplication provides a concise way to achieve this. However, for scenarios involving more complex operations or variations in element initialization, alternative approaches like loops or list comprehensions might be more suitable. Understanding the specific use case and the nature of the elements involved will help you determine whether list multiplication is the appropriate choice.
Nested Lists
While using list multiplication on flat lists is relatively straightforward, things can become more intricate when dealing with nested lists. If your list contains sublists or nested structures, you must carefully consider whether you want these nested structures to share references or have distinct copies. List multiplication applied to nested lists will repeat references at all levels, potentially leading to unintended changes across your data. Analyze your requirements and decide whether sharing references is acceptable or if you need separate instances for each nested element.
Code Readability
List multiplication can enhance code readability, especially when used to initialize lists with repeated values. However, excessive use of list multiplication can make your code more difficult to understand, particularly in situations where the number of repetitions is not immediately obvious. It’s essential to strike a balance between using list multiplication for clarity and avoiding overuse that might lead to confusion. If you find that your code’s intent isn’t immediately clear, consider adding comments to explain the purpose and reasoning behind your list multiplication operations, especially when dealing with complex operations or edge cases.
Conclusion
Python list multiplication is a valuable tool in a programmer’s toolkit. By understanding its mechanics and exploring its applications, you can enhance your code’s efficiency and readability. Whether you’re initializing lists, generating patterns, or manipulating strings, list multiplication provides a succinct and powerful solution.
So, the next time you find yourself needing to repeat elements in a list, consider leveraging the power of Python’s list multiplication to streamline your code and achieve your programming goals
FAQ
No, you cannot directly multiply lists of different lengths using the * operator. Python will raise a TypeError when attempting this operation.
Multiplying a list by 0 results in an empty list. For example, empty_list = [1, 2, 3] * 0 will yield an empty list [].
List multiplication involves repeating the same elements a certain number of times, while list concatenation involves combining two or more lists into a single list.
Yes, you can use negative values with list multiplication. However, using a negative multiplier will result in an empty list, similar to multiplying by 0.
No, list multiplication works with any type of element, not just integers and strings. You can multiply lists containing any data type.
For element-wise multiplication of lists containing numbers, you’ll need to use loops, list comprehensions, or libraries like NumPy. List multiplication itself doesn’t perform element-wise multiplication.