Unity has long been recognized as a leading game development platform, valued for its versatility and user-friendly interface. The engine offers an array of robust tools to help developers create interactive content, from small indie games to AAA titles. Among these tools, Unity’s Visual Scripting stands out as a unique and powerful feature.
What is Unity’s Visual Scripting?
Unity’s Visual Scripting is a feature that provides an intuitive, node-based system for coding behaviors into game objects without the need to write scripts manually in a text editor.
This tool makes the process of game development accessible to a wider range of creators, especially those who may not have a background in traditional text-based coding. It operates on a graphical interface where nodes, representing different functions or values, can be connected to create complex behaviors.
Advantages of Visual Scripting
- User-Friendly: Visual scripting eliminates the need for traditional text-based coding. It lowers the barrier to entry for new developers or those without a background in computer science.
- Time-Saving: The drag-and-drop interface makes prototyping faster, enabling developers to implement and test ideas more quickly.
- Collaboration-Friendly: Visual scripting can make the development process more collaborative, as it’s easier for non-programmers to understand and contribute to the project.
- Less Prone to Syntax Errors: Unlike text-based coding, which can be derailed by a misplaced character, visual scripting is less likely to encounter syntax errors.
Visual Scripting Workflow
The workflow in Unity’s visual scripting is centered around creating and connecting nodes in a graph. Below is a brief breakdown of the process:
Create a New Graph
Creating a new graph in Unity’s editor provides you with a canvas where you can develop your visual scripts. The graph serves as a visual representation of your script’s logic and allows you to easily manipulate and connect nodes to build the desired functionality. Here is a step-by-step guide on how to create a new graph in Unity:
Step | Description |
---|---|
1 | Open Unity’s editor and navigate to the desired location in your project where you want to create the new graph. |
2 | Right-click on the empty space within the project window or the desired folder, and select “Create” from the context menu. |
3 | In the submenu, choose “Visual Scripting” and then select the type of graph you want to create. Unity provides several options for graph types, such as State Machine Graph, Event Graph, or Behavior Graph. Select the one that suits your needs. |
4 | Unity will create a new graph asset in the selected location. By default, the graph asset will have a .asset extension and can be renamed according to your preference. |
5 | Double-click on the newly created graph asset to open it in the graph editor. |
6 | The graph editor interface will appear, displaying the canvas where you can build your visual scripts. The interface typically consists of a graph view, a toolbar, and a node palette. |
7 | Use the node palette to select and add nodes to the graph. Nodes represent different operations, variables, functions, or events that you can connect to define the logic of your script. You can usually find nodes organized into categories for easy navigation. |
8 | To add a node to the graph, simply click and drag it from the node palette onto the canvas. Position the node as desired. |
9 | Connect nodes together by clicking and dragging from the output port of one node to the input port of another. This establishes a connection between the nodes, allowing data or events to flow between them. |
10 | Customize the properties of nodes by selecting them and modifying their parameters in the inspector window. The inspector window provides access to the specific properties and settings of the selected node. |
11 | Continue adding nodes, connecting them, and configuring their properties to build your desired script’s logic. You can rearrange nodes on the canvas, zoom in or out, and pan the view to navigate and work on different parts of the graph. |
12 | Save your graph assets regularly to ensure your progress is preserved. You can either manually save by pressing Ctrl+S (Windows) or Command+S (Mac), or Unity may autosave your changes periodically. |
By following these steps, you can create a new graph in Unity’s editor and start building your visual scripts. The graph-based approach offers a visual and intuitive way to design your game logic, allowing for easier iteration and collaboration in the development process.
Add Nodes
In Unity’s graph editor, you can add nodes to your graph to represent various functions or values. Nodes serve as building blocks for your visual scripts and can be connected to define the logic and behavior of your game or application. Here are some details on adding nodes in Unity’s graph editor:
Types of Nodes | |
---|---|
Game Object Functions | These nodes represent actions that can be performed on game objects in your scene. Examples include “Move,” “Rotate,” “Destroy,” or “Spawn.” |
Mathematical Functions | These nodes allow you to perform mathematical operations such as addition, subtraction, multiplication, or division. |
Variables | Nodes representing variables hold data or values that can be used and manipulated within your graph. Examples include integers, floats, strings, or booleans. |
Flow Control | These nodes control the flow of execution within your graph, allowing you to branch, loop, or make decisions based on conditions. Examples include “If,” “While,” or “Switch” nodes. |
Adding Nodes | |
---|---|
Step 1 | Locate the node palette, usually positioned on the side or at the bottom of the graph editor interface. |
Step 2 | Browse through the available categories or search for specific nodes using the search bar within the node palette. |
Step 3 | Click and drag the desired node from the node palette onto the canvas of your graph. |
Step 4 | Position the node on the canvas as desired, arranging it in a way that makes the script logic clear and organized. |
Connecting Nodes | |
---|---|
Step 1 | Nodes can be connected to each other to establish data or event flow. |
Step 2 | Each node has input ports and output ports. Output ports produce data or events, while input ports receive them. |
Step 3 | To connect nodes, click and drag from the output port of one node to the input port of another. |
Step 4 | When the mouse is released, a connection will be created between the two nodes, indicating the flow of data or events. |
Customizing Node Properties | |
---|---|
Step 1 | Select a node on the canvas to display its properties and settings in the inspector window. |
Step 2 | In the inspector window, you can modify the specific parameters and properties of the selected node. |
Step 3 | Adjust the properties to suit your requirements and to fine-tune the behavior of the node within the graph. |
By adding nodes to your graph, you can create a visual representation of your script’s logic and functionality. Each node represents a specific function or value that contributes to the overall behavior of your game or application. Connecting nodes and customizing their properties allows you to define the flow of data and events, enabling you to create complex and interactive experiences within Unity.
Connect Nodes
Connecting nodes to define the logic of scripts is a fundamental concept in various fields like computer science, visual programming, game development, etc. In this context, a ‘node’ is a basic unit of a data structure, such as an object, a place, or a point in a network where information can be stored, received or transmitted. Let’s illustrate this process using a simple example where a ‘Move’ node and a ‘Time’ node are connected to create a script that moves an object over time.
The first step in connecting nodes is to understand the type of nodes we are dealing with:
- Move Node: This is a command that dictates the movement of an object from one place to another. It often takes parameters like distance and direction.
- Time Node: This node is related to the time taken to execute certain actions. It can be used to set the speed of the move or delay actions to a specific time.
To create a script that moves an object over time using the ‘Move’ and ‘Time’ nodes, follow these steps:
- Create nodes: Start by creating a ‘Move’ node and a ‘Time’ node.
Move Node | Time Node |
---|---|
Direction | Delay |
Distance | Duration |
- Set parameters: Define the specifics for each node, i.e., set the direction and distance for the ‘Move’ node and delay and duration for the ‘Time’ node.
Move Node | Time Node |
---|---|
Direction: North | Delay: 2 sec |
Distance: 10 m | Duration: 5 sec |
- Link nodes: Connect the ‘Move’ node to the ‘Time’ node. This forms a flow of command that allows the object to move in a specific direction for a certain distance over a defined time period.
Upon connecting the nodes as explained, we can develop a script. The script in pseudocode could look something like this:
BEGIN SCRIPT
DELAY 2 sec
MOVE object
DIRECTION North
DISTANCE 10 m
TIME 5 sec
END SCRIPT
When this script is executed, it will result in the following sequence:
- Wait for a delay of 2 seconds.
- Move the object to the North.
- Continue the movement for a distance of 10 meters.
- The entire movement should be completed in 5 seconds.
Remember, the understanding of nodes and their properties can significantly differ based on the system or language you are using. Always make sure to understand the specific context you are working in to properly connect nodes and create functional scripts.
Table: Common Node Types and Functions
Node Type | Function |
---|---|
Event | Trigger an action |
Flow | Control the execution of the script |
Variable | Store and manipulate data |
Function | Perform specific tasks |
Visual Scripting Applications
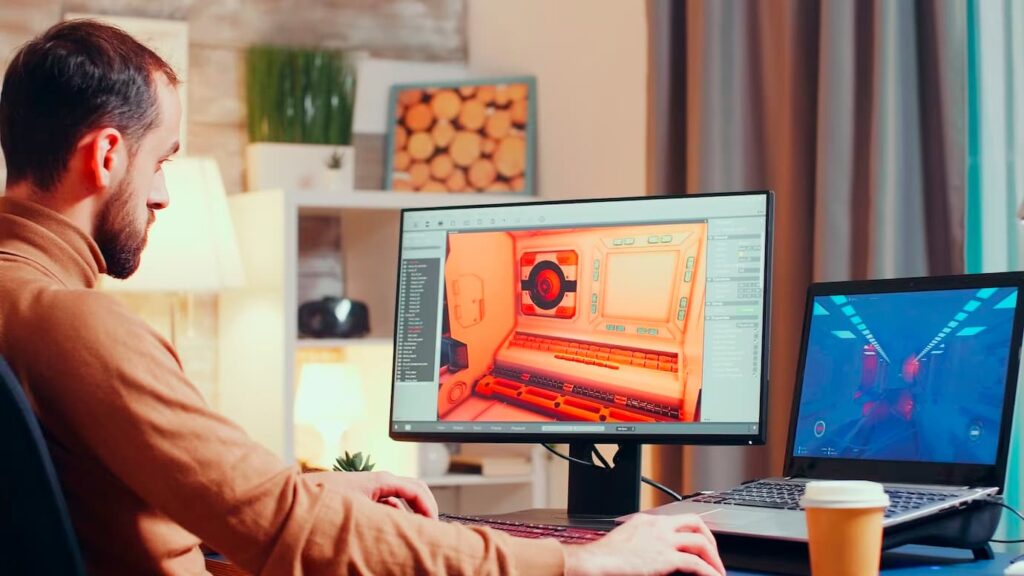
Visual scripting is a powerful tool within the Unity engine that allows developers to create complex interactions, behaviors, and mechanics without writing traditional code. It offers a more intuitive and accessible approach to game development, making it ideal for both beginners and experienced programmers. In this article, we will explore various applications of visual scripting and how they can be used to enhance game development in Unity.
- Character Movement: Visual scripting can be used to control character movements, animations, and interactions with the environment. With visual scripting, developers can easily create and modify character movement mechanics, such as walking, running, jumping, and crouching. They can also implement advanced features like ledge grabbing, wall running, or swimming. By visually connecting nodes representing different actions and conditions, developers can define the logic behind character movement with ease.
- AI Behavior: One of the major applications of visual scripting is prototyping and testing AI behavior patterns. Developers can visually design and modify AI behaviors, ranging from simple enemy movement patterns to complex decision-making processes. Visual scripting allows for the creation of AI agents that can react to the player’s actions, navigate the game world intelligently, and engage in combat or other interactions. This streamlines the process of iterating on AI behaviors, making it easier to fine-tune and balance enemy encounters.
- Game Mechanics: Visual scripting can be utilized to code fundamental game mechanics, adding interactivity and depth to the gameplay experience. Developers can create scoring systems, power-ups, level transitions, puzzles, quests, and much more using visual scripting tools. By designing visual scripts, they can define the rules and conditions that govern these mechanics. For example, they can set up triggers that activate certain events or modify variables that affect the game state. Visual scripting provides a flexible and efficient way to implement game mechanics without extensive coding.
- Environmental Interactions: Visual scripting can manage the interactive components of a game’s environment, allowing developers to create dynamic and responsive worlds. For instance, developers can use visual scripting to trigger animations or play sounds when the player interacts with objects in the game. This includes actions like opening doors, activating switches, breaking objects, or causing environmental effects. With visual scripting, developers can easily define the cause-and-effect relationships between player actions and environmental responses.
Conclusion
Unity’s Visual Scripting is a robust tool that democratizes the game development process. Whether you’re a beginner hoping to dive into the world of game creation or a seasoned developer looking to streamline your workflow, visual scripting can offer a wealth of benefits. It provides a user-friendly, time-efficient way to implement intricate mechanics and behaviors in your Unity projects, making it an essential part of any game developer’s toolkit.
FAQ
Q1: Can I use visual scripting for my entire project, or do I still need to code?
While it’s possible to build a game using only visual scripting, larger and more complex projects may still require traditional coding for certain aspects. However, visual scripting can significantly reduce the amount of manual coding needed.
Q2: How does visual scripting affect performance?
Visual scripting is slightly less performant than traditional coding due to the overhead of the graphical interface. However, for most small to medium-sized projects, the difference is negligible.
Q3: Can I convert my visual scripts into C# code?
As of the last update in 2023, Unity does not support converting visual scripts directly into C# code. You can, however, use the visual scripting as a prototyping tool before implementing the logic in C#.
Q4: Do I need to understand coding principles to use visual scripting?
While visual scripting eliminates the need for writing code manually, it’s still beneficial to understand basic programming principles. Concepts like loops, variables, and conditionals are still relevant in the visual scripting environment.